Integration when using Stripe Elements for checkout
Overview
Stigg allows you to implement custom checkout experiences using Stripe Elements, while delegating the subscription lifecycle management to Stigg.
Doing so will require the following process:
- Collection of the payment method using Stripe Elements
- Updating the customer in Stigg using the Stigg server SDKs
- Provisioning or updating the subscription in Stigg using the Stigg server SDKs
Stigg will then auto-magically create and update the customer and subscription objects in Stripe π§πͺ
Before we begin
In order to complete this guide in your application code, please make sure that you have:
- Access to a Stripe account
- A Stigg environment that's integrated with Stripe
- Modeled your pricing in Stigg
- Know how to provision customers in Stigg
- Know how to update subscriptions in Stigg
How it works
It's typical to build the checkout form using Stripe.js elements and leverage the Setup Intents API to confirm the payment method and save it for future use.
If you need help in setting this up, you can refer to this article to learn how setup intents work.
In case you have a different setup in place, let us know and we'll assist you with the integration to suit your needs.
Once your checkout form is built, the journey of the customer should look similar to this one:
Provision a new subscription
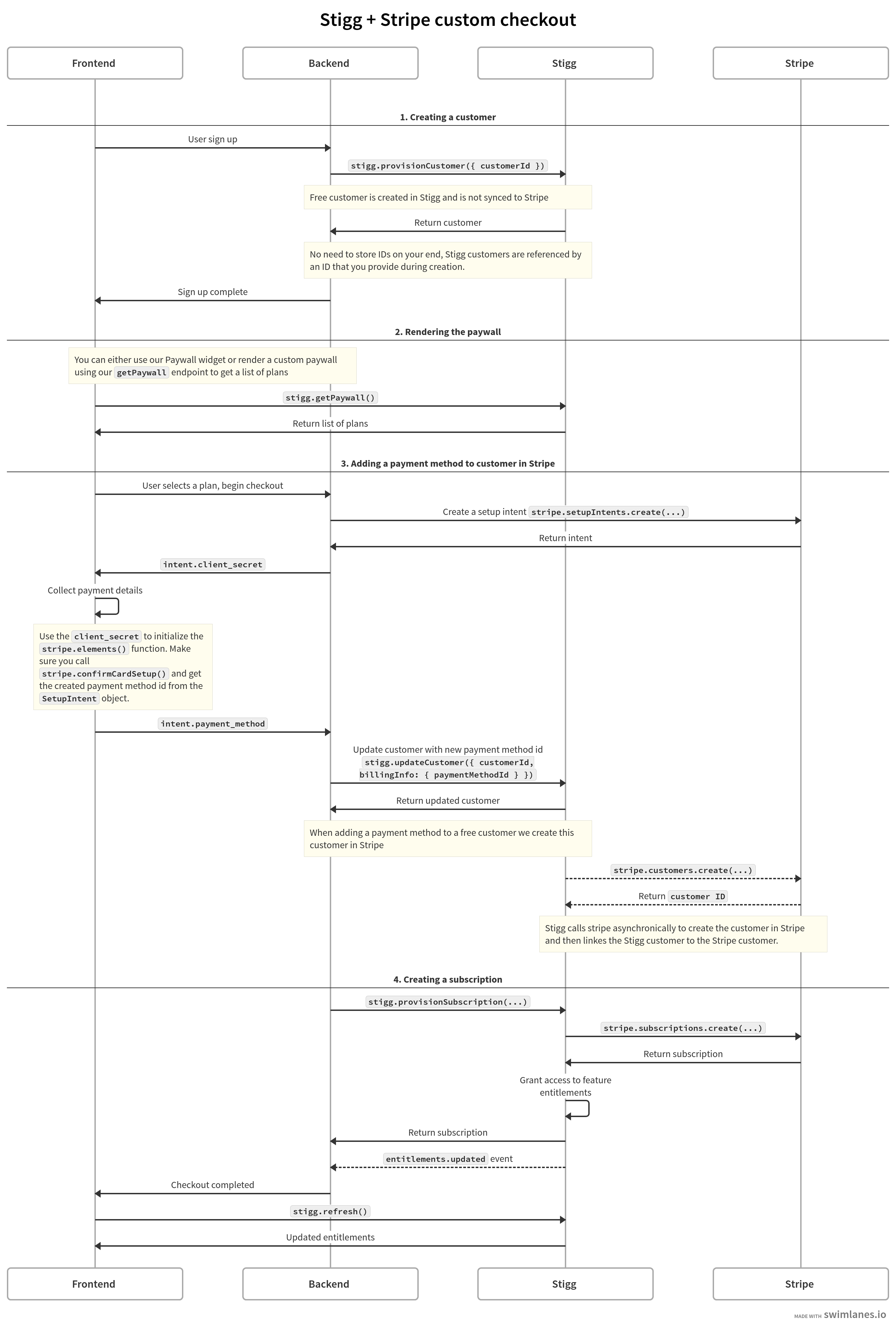
Update an existing subscription
Provisioning a customer
When a new user sign up to your application, provision a customer in Stigg to represent an individual or an organization:
await stiggClient.provisionCustomer({
customerId: 'CUSTOMER-ID',
subscriptionParams: null,
...
});
Rendering the paywall
To render a paywall inside your application, you can use Stigg's embeddable widget or render your own pricing plans component based on the data fetched from Stigg:
Adding a payment method to the customer in Stripe
When a user selects a plan, present the custom checkout form to add the new payment method.
According to official Stripe documentation, you should create the SetupIntent
for the customer on your backend, and then propagate the returned intent client_secret
to the frontend.
It can then be used to initialize the stripe.elements
form in a secured manner.
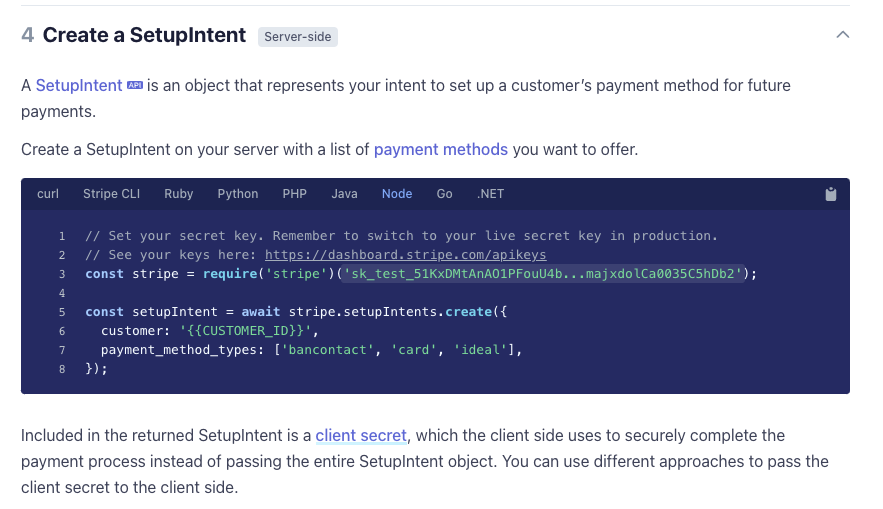
Creating a SetupIntent
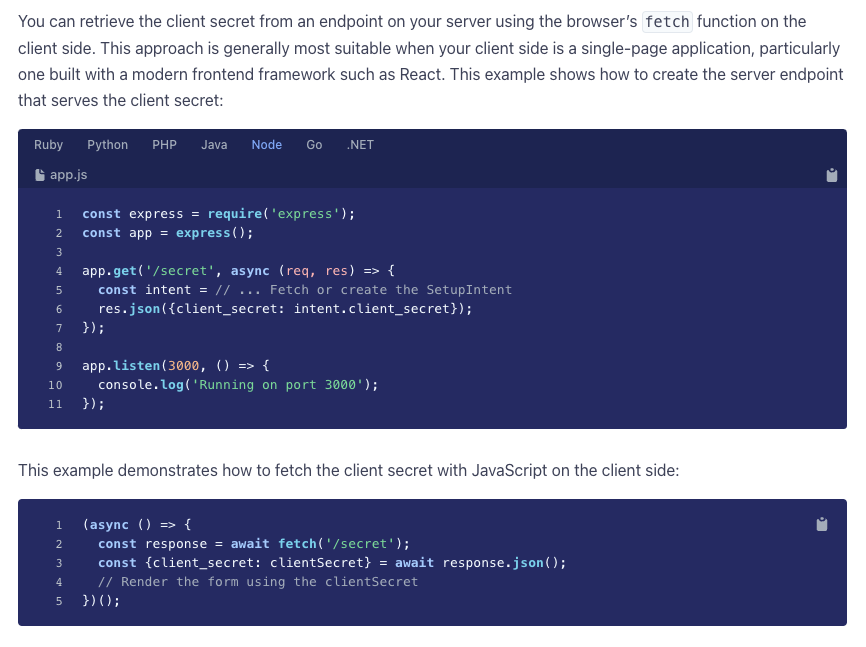
Exposing the SetupIntent to the client
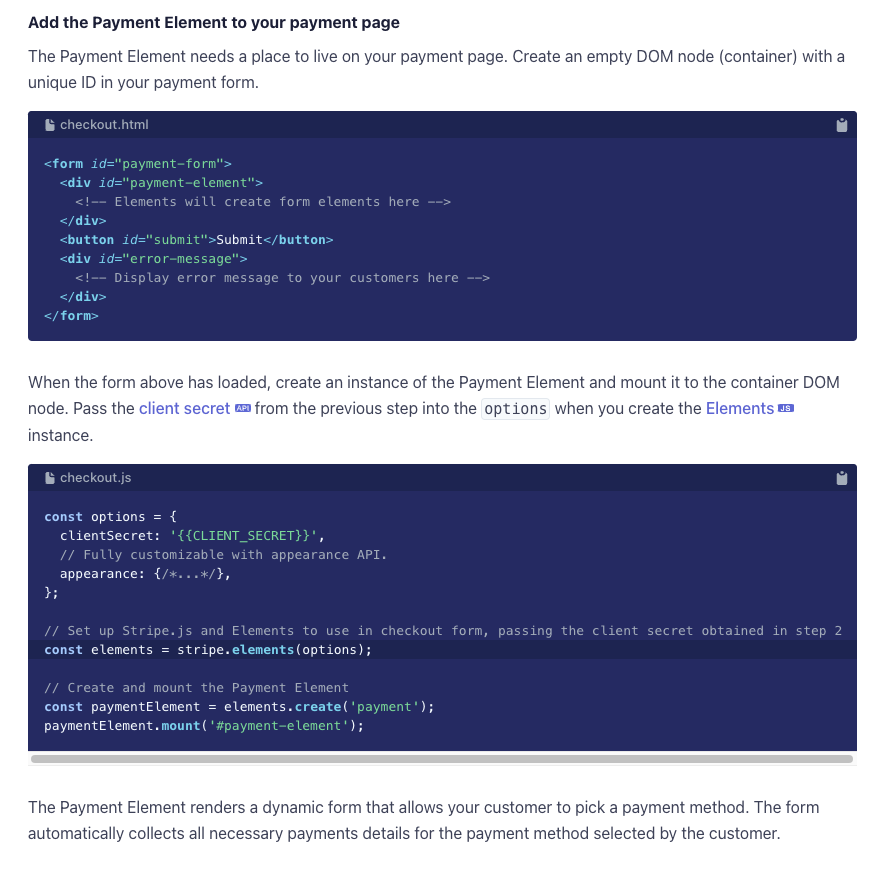
Provide the client_secret
to stripe.elements()
options
The checkout form should handle the validation of the card prior creating subscription in Stigg, and once its valid you will get a payment_method
id on the return value.
You can attach the newly created payment method to a customer in Stigg using by passing the returned payment_method
ID to the updateCustomer
method.
await stiggClient.updateCustomer({
customerId: 'CUSTOMER-ID',
billingInfo: { paymentMethodId: 'PAYMENT-METHOD-ID'}
})
Provisioning a subscription
After attaching the payment method to the customer, provision a subscription in Stigg:
const subscription = await stiggClient.provisionSubscription({
customerId: 'CUSTOMER-ID',
planId: 'PLAN-ID',
...
})
At this point, Stigg will attempt to create a subscription in Stripe and use the default payment method to be charged for the generated invoice in the case of a paid plan.
Handling successful payments
Once the payment is successful, the subscription will become active in Stigg and the customer will be granted access to the associated entitlements.
On the front-end, you should call the refresh
function to re-fetch the current customer data and the new entitlements.
await stiggClient.refresh();
Stigg will update the server SDKs in real-time by pushing the updates over a web-socket connection.
You can also optionally subscribe to to the entitlements.updated
webhook event to update third-party applications.
Handling failed payments
If the payment was not successful or requires additional steps (for example: when 3DS confirmation is required), the provisionSubscription
method will return an appropriate response.
Stripe creates a time frame (default of 24 hours) for the outstanding invoice to be settled. If the invoice is not settled within that time frame, the subscription is canceled both in Stripe and Stigg.
Handling failure of recurring payments
You can notify customers about failed payments by listening to Stigg's customer.payment_failed
webhook. The customer should be instructed to replace the payment method with a different one and to try again.
Updated about 1 year ago